forked from javadev/LeetCode-in-Java
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
15 changed files
with
553 additions
and
0 deletions.
There are no files selected for viewing
40 changes: 40 additions & 0 deletions
40
src/main/java/g3001_3100/s3026_maximum_good_subarray_sum/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
package g3001_3100.s3026_maximum_good_subarray_sum; | ||
|
||
// #Medium #Array #Hash_Table #Prefix_Sum #2024_03_01_Time_62_ms_(93.52%)_Space_56.2_MB_(95.77%) | ||
|
||
import java.util.HashMap; | ||
import java.util.Map; | ||
|
||
public class Solution { | ||
private static final int NO_GOOD_SUBARRAYS = 0; | ||
|
||
public long maximumSubarraySum(int[] input, int targetDifference) { | ||
Map<Integer, Long> valueToMinPrefixSum = new HashMap<>(); | ||
long prefixSum = 0; | ||
long maxSubarraySum = Long.MIN_VALUE; | ||
for (int value : input) { | ||
if (valueToMinPrefixSum.containsKey(value + targetDifference)) { | ||
maxSubarraySum = | ||
Math.max( | ||
maxSubarraySum, | ||
prefixSum | ||
+ value | ||
- valueToMinPrefixSum.get(value + targetDifference)); | ||
} | ||
if (valueToMinPrefixSum.containsKey(value - targetDifference)) { | ||
maxSubarraySum = | ||
Math.max( | ||
maxSubarraySum, | ||
prefixSum | ||
+ value | ||
- valueToMinPrefixSum.get(value - targetDifference)); | ||
} | ||
if (!valueToMinPrefixSum.containsKey(value) | ||
|| valueToMinPrefixSum.get(value) > prefixSum) { | ||
valueToMinPrefixSum.put(value, prefixSum); | ||
} | ||
prefixSum += value; | ||
} | ||
return maxSubarraySum != Long.MIN_VALUE ? maxSubarraySum : NO_GOOD_SUBARRAYS; | ||
} | ||
} |
39 changes: 39 additions & 0 deletions
39
src/main/java/g3001_3100/s3026_maximum_good_subarray_sum/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,39 @@ | ||
3026\. Maximum Good Subarray Sum | ||
|
||
Medium | ||
|
||
You are given an array `nums` of length `n` and a **positive** integer `k`. | ||
|
||
A subarray of `nums` is called **good** if the **absolute difference** between its first and last element is **exactly** `k`, in other words, the subarray `nums[i..j]` is good if `|nums[i] - nums[j]| == k`. | ||
|
||
Return _the **maximum** sum of a **good** subarray of_ `nums`. _If there are no good subarrays__, return_ `0`. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [1,2,3,4,5,6], k = 1 | ||
|
||
**Output:** 11 | ||
|
||
**Explanation:** The absolute difference between the first and last element must be 1 for a good subarray. All the good subarrays are: [1,2], [2,3], [3,4], [4,5], and [5,6]. The maximum subarray sum is 11 for the subarray [5,6]. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [-1,3,2,4,5], k = 3 | ||
|
||
**Output:** 11 | ||
|
||
**Explanation:** The absolute difference between the first and last element must be 3 for a good subarray. All the good subarrays are: [-1,3,2], and [2,4,5]. The maximum subarray sum is 11 for the subarray [2,4,5]. | ||
|
||
**Example 3:** | ||
|
||
**Input:** nums = [-1,-2,-3,-4], k = 2 | ||
|
||
**Output:** -6 | ||
|
||
**Explanation:** The absolute difference between the first and last element must be 2 for a good subarray. All the good subarrays are: [-1,-2,-3], and [-2,-3,-4]. The maximum subarray sum is -6 for the subarray [-1,-2,-3]. | ||
|
||
**Constraints:** | ||
|
||
* <code>2 <= nums.length <= 10<sup>5</sup></code> | ||
* <code>-10<sup>9</sup> <= nums[i] <= 10<sup>9</sup></code> | ||
* <code>1 <= k <= 10<sup>9</sup></code> |
30 changes: 30 additions & 0 deletions
30
src/main/java/g3001_3100/s3027_find_the_number_of_ways_to_place_people_ii/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
package g3001_3100.s3027_find_the_number_of_ways_to_place_people_ii; | ||
|
||
// #Hard #Array #Math #Sorting #Enumeration #Geometry | ||
// #2024_03_01_Time_59_ms_(69.95%)_Space_45.3_MB_(20.75%) | ||
|
||
import java.util.Arrays; | ||
|
||
public class Solution { | ||
private int customCompare(int[] p1, int[] p2) { | ||
if (p1[0] != p2[0]) { | ||
return Integer.signum(p1[0] - p2[0]); | ||
} | ||
return Integer.signum(p2[1] - p1[1]); | ||
} | ||
|
||
public int numberOfPairs(int[][] points) { | ||
Arrays.sort(points, this::customCompare); | ||
int count = 0; | ||
for (int i = 0; i < points.length; ++i) { | ||
int m = Integer.MIN_VALUE; | ||
for (int j = i + 1; j < points.length; ++j) { | ||
if ((points[i][1] >= points[j][1]) && (points[j][1] > m)) { | ||
m = points[j][1]; | ||
count++; | ||
} | ||
} | ||
} | ||
return count; | ||
} | ||
} |
63 changes: 63 additions & 0 deletions
63
...main/java/g3001_3100/s3027_find_the_number_of_ways_to_place_people_ii/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,63 @@ | ||
3027\. Find the Number of Ways to Place People II | ||
|
||
Hard | ||
|
||
You are given a 2D array `points` of size `n x 2` representing integer coordinates of some points on a 2D-plane, where <code>points[i] = [x<sub>i</sub>, y<sub>i</sub>]</code>. | ||
|
||
We define the **right** direction as positive x-axis (**increasing x-coordinate**) and the **left** direction as negative x-axis (**decreasing x-coordinate**). Similarly, we define the **up** direction as positive y-axis (**increasing y-coordinate**) and the **down** direction as negative y-axis (**decreasing y-coordinate**) | ||
|
||
You have to place `n` people, including Alice and Bob, at these points such that there is **exactly one** person at every point. Alice wants to be alone with Bob, so Alice will build a rectangular fence with Alice's position as the **upper left corner** and Bob's position as the **lower right corner** of the fence (**Note** that the fence **might not** enclose any area, i.e. it can be a line). If any person other than Alice and Bob is either **inside** the fence or **on** the fence, Alice will be sad. | ||
|
||
Return _the number of **pairs of points** where you can place Alice and Bob, such that Alice **does not** become sad on building the fence_. | ||
|
||
**Note** that Alice can only build a fence with Alice's position as the upper left corner, and Bob's position as the lower right corner. For example, Alice cannot build either of the fences in the picture below with four corners `(1, 1)`, `(1, 3)`, `(3, 1)`, and `(3, 3)`, because: | ||
|
||
* With Alice at `(3, 3)` and Bob at `(1, 1)`, Alice's position is not the upper left corner and Bob's position is not the lower right corner of the fence. | ||
* With Alice at `(1, 3)` and Bob at `(1, 1)`, Bob's position is not the lower right corner of the fence. | ||
|
||
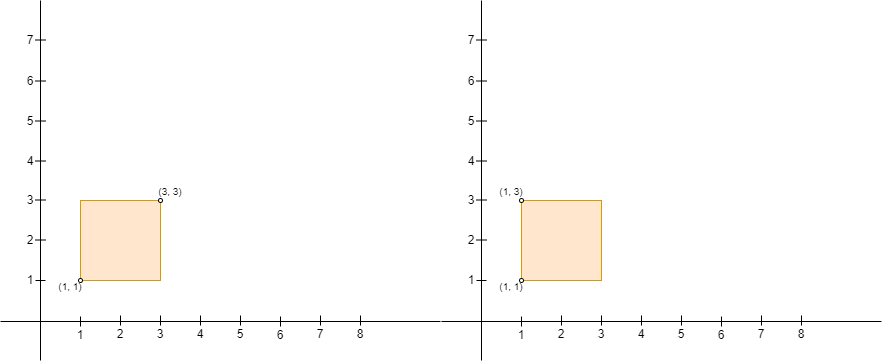 | ||
|
||
**Example 1:** | ||
|
||
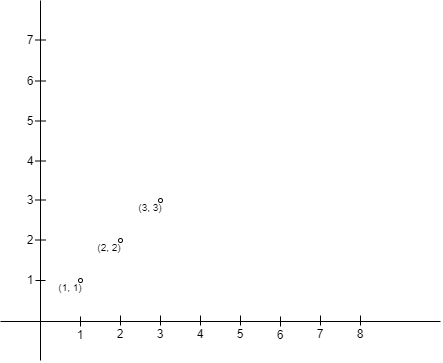 | ||
|
||
**Input:** points = [[1,1],[2,2],[3,3]] | ||
|
||
**Output:** 0 | ||
|
||
**Explanation:** There is no way to place Alice and Bob such that Alice can build a fence with Alice's position as the upper left corner and Bob's position as the lower right corner. Hence we return 0. | ||
|
||
**Example 2:** | ||
|
||
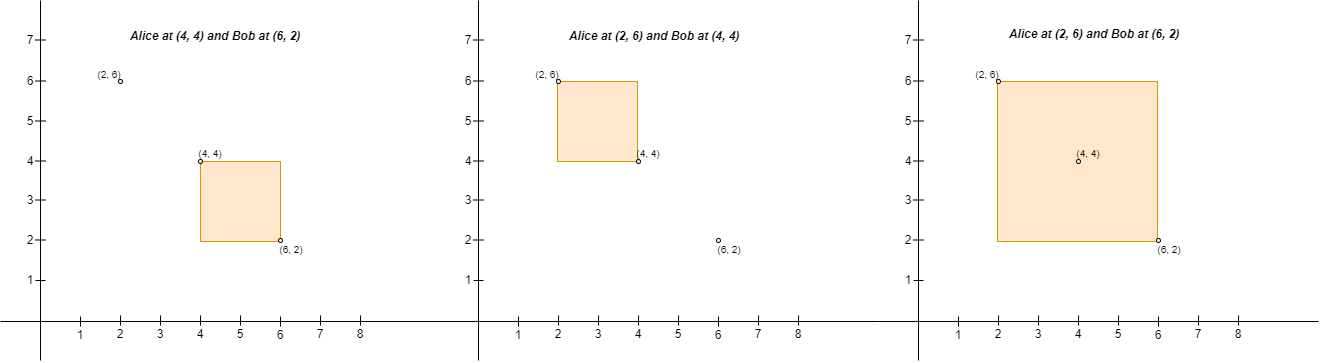 | ||
|
||
**Input:** points = [[6,2],[4,4],[2,6]] | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** There are two ways to place Alice and Bob such that Alice will not be sad: | ||
- Place Alice at (4, 4) and Bob at (6, 2). | ||
- Place Alice at (2, 6) and Bob at (4, 4). You cannot place Alice at (2, 6) and Bob at (6, 2) because the person at (4, 4) will be inside the fence. | ||
|
||
**Example 3:** | ||
|
||
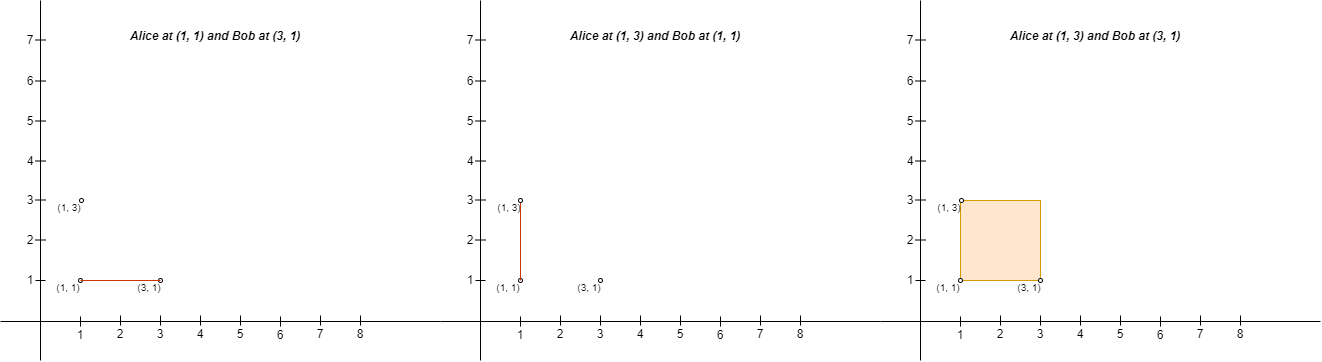 | ||
|
||
**Input:** points = [[3,1],[1,3],[1,1]] | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** There are two ways to place Alice and Bob such that Alice will not be sad: | ||
- Place Alice at (1, 1) and Bob at (3, 1). | ||
- Place Alice at (1, 3) and Bob at (1, 1). | ||
|
||
You cannot place Alice at (1, 3) and Bob at (3, 1) because the person at (1, 1) will be on the fence. | ||
|
||
Note that it does not matter if the fence encloses any area, the first and second fences in the image are valid. | ||
|
||
**Constraints:** | ||
|
||
* `2 <= n <= 1000` | ||
* `points[i].length == 2` | ||
* <code>-10<sup>9</sup> <= points[i][0], points[i][1] <= 10<sup>9</sup></code> | ||
* All `points[i]` are distinct. |
17 changes: 17 additions & 0 deletions
17
src/main/java/g3001_3100/s3028_ant_on_the_boundary/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
package g3001_3100.s3028_ant_on_the_boundary; | ||
|
||
// #Easy #Array #Simulation #Prefix_Sum #2024_03_01_Time_0_ms_(100.00%)_Space_42.1_MB_(53.10%) | ||
|
||
public class Solution { | ||
public int returnToBoundaryCount(int[] nums) { | ||
int ans = 0; | ||
int num = 0; | ||
for (int n : nums) { | ||
num += n; | ||
if (num == 0) { | ||
ans++; | ||
} | ||
} | ||
return ans; | ||
} | ||
} |
51 changes: 51 additions & 0 deletions
51
src/main/java/g3001_3100/s3028_ant_on_the_boundary/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,51 @@ | ||
3028\. Ant on the Boundary | ||
|
||
Easy | ||
|
||
An ant is on a boundary. It sometimes goes **left** and sometimes **right**. | ||
|
||
You are given an array of **non-zero** integers `nums`. The ant starts reading `nums` from the first element of it to its end. At each step, it moves according to the value of the current element: | ||
|
||
* If `nums[i] < 0`, it moves **left** by `-nums[i]` units. | ||
* If `nums[i] > 0`, it moves **right** by `nums[i]` units. | ||
|
||
Return _the number of times the ant **returns** to the boundary._ | ||
|
||
**Notes:** | ||
|
||
* There is an infinite space on both sides of the boundary. | ||
* We check whether the ant is on the boundary only after it has moved `|nums[i]|` units. In other words, if the ant crosses the boundary during its movement, it does not count. | ||
|
||
**Example 1:** | ||
|
||
**Input:** nums = [2,3,-5] | ||
|
||
**Output:** 1 | ||
|
||
**Explanation:** After the first step, the ant is 2 steps to the right of the boundary. | ||
|
||
After the second step, the ant is 5 steps to the right of the boundary. | ||
|
||
After the third step, the ant is on the boundary. So the answer is 1. | ||
|
||
**Example 2:** | ||
|
||
**Input:** nums = [3,2,-3,-4] | ||
|
||
**Output:** 0 | ||
|
||
**Explanation:** After the first step, the ant is 3 steps to the right of the boundary. | ||
|
||
After the second step, the ant is 5 steps to the right of the boundary. | ||
|
||
After the third step, the ant is 2 steps to the right of the boundary. | ||
|
||
After the fourth step, the ant is 2 steps to the left of the boundary. | ||
|
||
The ant never returned to the boundary, so the answer is 0. | ||
|
||
**Constraints:** | ||
|
||
* `1 <= nums.length <= 100` | ||
* `-10 <= nums[i] <= 10` | ||
* `nums[i] != 0` |
16 changes: 16 additions & 0 deletions
16
src/main/java/g3001_3100/s3029_minimum_time_to_revert_word_to_initial_state_i/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
package g3001_3100.s3029_minimum_time_to_revert_word_to_initial_state_i; | ||
|
||
// #Medium #String #Hash_Function #String_Matching #Rolling_Hash | ||
// #2024_03_01_Time_1_ms_(99.70%)_Space_42.7_MB_(8.42%) | ||
|
||
public class Solution { | ||
public int minimumTimeToInitialState(String word, int k) { | ||
int n = word.length(); | ||
for (int i = k; i < n; i += k) { | ||
if (word.substring(i, n).equals(word.substring(0, n - i))) { | ||
return i / k; | ||
} | ||
} | ||
return (n + k - 1) / k; | ||
} | ||
} |
44 changes: 44 additions & 0 deletions
44
.../java/g3001_3100/s3029_minimum_time_to_revert_word_to_initial_state_i/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,44 @@ | ||
3029\. Minimum Time to Revert Word to Initial State I | ||
|
||
Medium | ||
|
||
You are given a **0-indexed** string `word` and an integer `k`. | ||
|
||
At every second, you must perform the following operations: | ||
|
||
* Remove the first `k` characters of `word`. | ||
* Add any `k` characters to the end of `word`. | ||
|
||
**Note** that you do not necessarily need to add the same characters that you removed. However, you must perform **both** operations at every second. | ||
|
||
Return _the **minimum** time greater than zero required for_ `word` _to revert to its **initial** state_. | ||
|
||
**Example 1:** | ||
|
||
**Input:** word = "abacaba", k = 3 | ||
|
||
**Output:** 2 | ||
|
||
**Explanation:** At the 1st second, we remove characters "aba" from the prefix of word, and add characters "bac" to the end of word. Thus, word becomes equal to "cababac". At the 2nd second, we remove characters "cab" from the prefix of word, and add "aba" to the end of word. Thus, word becomes equal to "abacaba" and reverts to its initial state. It can be shown that 2 seconds is the minimum time greater than zero required for word to revert to its initial state. | ||
|
||
**Example 2:** | ||
|
||
**Input:** word = "abacaba", k = 4 | ||
|
||
**Output:** 1 | ||
|
||
**Explanation:** At the 1st second, we remove characters "abac" from the prefix of word, and add characters "caba" to the end of word. Thus, word becomes equal to "abacaba" and reverts to its initial state. It can be shown that 1 second is the minimum time greater than zero required for word to revert to its initial state. | ||
|
||
**Example 3:** | ||
|
||
**Input:** word = "abcbabcd", k = 2 | ||
|
||
**Output:** 4 | ||
|
||
**Explanation:** At every second, we will remove the first 2 characters of word, and add the same characters to the end of word. After 4 seconds, word becomes equal to "abcbabcd" and reverts to its initial state. It can be shown that 4 seconds is the minimum time greater than zero required for word to revert to its initial state. | ||
|
||
**Constraints:** | ||
|
||
* `1 <= word.length <= 50` | ||
* `1 <= k <= word.length` | ||
* `word` consists only of lowercase English letters. |
65 changes: 65 additions & 0 deletions
65
src/main/java/g3001_3100/s3030_find_the_grid_of_region_average/Solution.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,65 @@ | ||
package g3001_3100.s3030_find_the_grid_of_region_average; | ||
|
||
// #Medium #Array #Matrix #2024_03_01_Time_53_ms_(98.79%)_Space_88.4_MB_(35.20%) | ||
|
||
public class Solution { | ||
public int[][] resultGrid(int[][] image, int threshold) { | ||
int n = image.length; | ||
int m = image[0].length; | ||
int[][] intensity = new int[n][m]; | ||
int[][] count = new int[n][m]; | ||
for (int i = 1; i < n - 1; i++) { | ||
for (int j = 1; j < m - 1; j++) { | ||
boolean regionPossible = true; | ||
int regionSum = 0; | ||
int r0c0 = image[i - 1][j - 1]; | ||
int r0c1 = image[i - 1][j]; | ||
int r0c2 = image[i - 1][j + 1]; | ||
int r1c0 = image[i][j - 1]; | ||
int r1c1 = image[i][j]; | ||
int r1c2 = image[i][j + 1]; | ||
int r2c0 = image[i + 1][j - 1]; | ||
int r2c1 = image[i + 1][j]; | ||
int r2c2 = image[i + 1][j + 1]; | ||
regionSum += (r0c0 + r0c1 + r0c2 + r1c0 + r1c1 + r1c2 + r2c0 + r2c1 + r2c2); | ||
if (Math.abs(r0c0 - r0c1) > threshold | ||
|| Math.abs(r0c0 - r1c0) > threshold | ||
|| Math.abs(r0c1 - r0c0) > threshold | ||
|| Math.abs(r0c1 - r1c1) > threshold | ||
|| Math.abs(r0c1 - r0c2) > threshold | ||
|| Math.abs(r0c2 - r0c1) > threshold | ||
|| Math.abs(r0c2 - r1c2) > threshold | ||
|| Math.abs(r1c0 - r1c1) > threshold | ||
|| Math.abs(r1c2 - r1c1) > threshold | ||
|| Math.abs(r2c0 - r2c1) > threshold | ||
|| Math.abs(r2c0 - r1c0) > threshold | ||
|| Math.abs(r2c1 - r2c0) > threshold | ||
|| Math.abs(r2c1 - r1c1) > threshold | ||
|| Math.abs(r2c1 - r2c2) > threshold | ||
|| Math.abs(r2c2 - r2c1) > threshold | ||
|| Math.abs(r2c2 - r1c2) > threshold) { | ||
regionPossible = false; | ||
} | ||
if (regionPossible) { | ||
regionSum /= 9; | ||
for (int k = -1; k <= 1; k++) { | ||
for (int l = -1; l <= 1; l++) { | ||
intensity[i + k][j + l] += regionSum; | ||
count[i + k][j + l]++; | ||
} | ||
} | ||
} | ||
} | ||
} | ||
for (int i = 0; i < n; i++) { | ||
for (int j = 0; j < m; j++) { | ||
if (count[i][j] == 0) { | ||
intensity[i][j] = image[i][j]; | ||
} else { | ||
intensity[i][j] = intensity[i][j] / count[i][j]; | ||
} | ||
} | ||
} | ||
return intensity; | ||
} | ||
} |
Oops, something went wrong.