forked from LeetCode-in-Php/LeetCode-in-Php
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
33 changed files
with
984 additions
and
3 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,43 @@ | ||
<?php | ||
|
||
namespace leetcode\g0101_0200\s0101_symmetric_tree; | ||
|
||
// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Depth_First_Search #Breadth_First_Search | ||
// #Tree #Binary_Tree #Data_Structure_I_Day_11_Tree #Level_2_Day_15_Tree | ||
// #Big_O_Time_O(N)_Space_O(log(N)) #2023_12_11_Time_6_ms_(76.40%)_Space_19.4_MB_(28.09%) | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* class TreeNode { | ||
* public $val = null; | ||
* public $left = null; | ||
* public $right = null; | ||
* function __construct($val = 0, $left = null, $right = null) { | ||
* $this->val = $val; | ||
* $this->left = $left; | ||
* $this->right = $right; | ||
* } | ||
* } | ||
*/ | ||
class Solution { | ||
/** | ||
* @param TreeNode $root | ||
* @return Boolean | ||
*/ | ||
function isSymmetric($root) { | ||
if ($root == null) { | ||
return true; | ||
} | ||
return $this->helper($root->left, $root->right); | ||
} | ||
|
||
private function helper($leftNode, $rightNode) { | ||
if ($leftNode == null || $rightNode == null) { | ||
return $leftNode == null && $rightNode == null; | ||
} | ||
if ($leftNode->val != $rightNode->val) { | ||
return false; | ||
} | ||
return $this->helper($leftNode->left, $rightNode->right) && $this->helper($leftNode->right, $rightNode->left); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
101\. Symmetric Tree | ||
|
||
Easy | ||
|
||
Given the `root` of a binary tree, _check whether it is a mirror of itself_ (i.e., symmetric around its center). | ||
|
||
**Example 1:** | ||
|
||
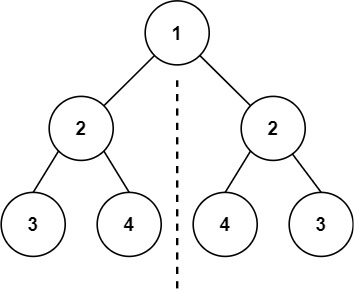 | ||
|
||
**Input:** root = [1,2,2,3,4,4,3] | ||
|
||
**Output:** true | ||
|
||
**Example 2:** | ||
|
||
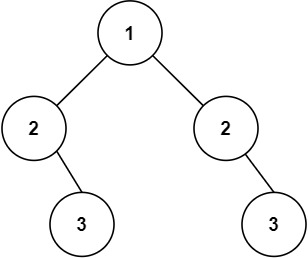 | ||
|
||
**Input:** root = [1,2,2,null,3,null,3] | ||
|
||
**Output:** false | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is in the range `[1, 1000]`. | ||
* `-100 <= Node.val <= 100` | ||
|
||
**Follow up:** Could you solve it both recursively and iteratively? |
56 changes: 56 additions & 0 deletions
56
src/main/php/g0101_0200/s0102_binary_tree_level_order_traversal/Solution.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,56 @@ | ||
<?php | ||
|
||
namespace leetcode\g0101_0200\s0102_binary_tree_level_order_traversal; | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Breadth_First_Search #Tree | ||
// #Binary_Tree #Data_Structure_I_Day_11_Tree #Level_1_Day_6_Tree #Udemy_Tree_Stack_Queue | ||
// #Big_O_Time_O(N)_Space_O(N) #2023_12_11_Time_4_ms_(96.08%)_Space_20.9_MB_(50.98%) | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* class TreeNode { | ||
* public $val = null; | ||
* public $left = null; | ||
* public $right = null; | ||
* function __construct($val = 0, $left = null, $right = null) { | ||
* $this->val = $val; | ||
* $this->left = $left; | ||
* $this->right = $right; | ||
* } | ||
* } | ||
*/ | ||
class Solution { | ||
/** | ||
* @param TreeNode $root | ||
* @return Integer[][] | ||
*/ | ||
function levelOrder($root) { | ||
$result = array(); | ||
if ($root == null) { | ||
return $result; | ||
} | ||
$queue = new \SplQueue(); | ||
$queue->enqueue($root); | ||
$queue->enqueue(null); | ||
$level = array(); | ||
while (!$queue->isEmpty()) { | ||
$root = $queue->dequeue(); | ||
while (!$queue->isEmpty() && $root != null) { | ||
array_push($level, $root->val); | ||
if ($root->left != null) { | ||
$queue->enqueue($root->left); | ||
} | ||
if ($root->right != null) { | ||
$queue->enqueue($root->right); | ||
} | ||
$root = $queue->dequeue(); | ||
} | ||
array_push($result, $level); | ||
$level = array(); | ||
if (!$queue->isEmpty()) { | ||
$queue->enqueue(null); | ||
} | ||
} | ||
return $result; | ||
} | ||
} |
30 changes: 30 additions & 0 deletions
30
src/main/php/g0101_0200/s0102_binary_tree_level_order_traversal/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
102\. Binary Tree Level Order Traversal | ||
|
||
Medium | ||
|
||
Given the `root` of a binary tree, return _the level order traversal of its nodes' values_. (i.e., from left to right, level by level). | ||
|
||
**Example 1:** | ||
|
||
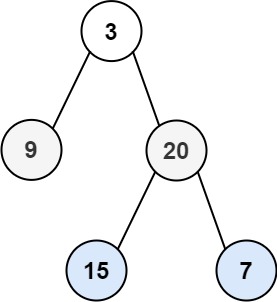 | ||
|
||
**Input:** root = [3,9,20,null,null,15,7] | ||
|
||
**Output:** [[3],[9,20],[15,7]] | ||
|
||
**Example 2:** | ||
|
||
**Input:** root = [1] | ||
|
||
**Output:** [[1]] | ||
|
||
**Example 3:** | ||
|
||
**Input:** root = [] | ||
|
||
**Output:** [] | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is in the range `[0, 2000]`. | ||
* `-1000 <= Node.val <= 1000` |
40 changes: 40 additions & 0 deletions
40
src/main/php/g0101_0200/s0104_maximum_depth_of_binary_tree/Solution.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
<?php | ||
|
||
namespace leetcode\g0101_0200\s0104_maximum_depth_of_binary_tree; | ||
|
||
// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Depth_First_Search #Breadth_First_Search | ||
// #Tree #Binary_Tree #Data_Structure_I_Day_11_Tree | ||
// #Programming_Skills_I_Day_10_Linked_List_and_Tree #Udemy_Tree_Stack_Queue | ||
// #Big_O_Time_O(N)_Space_O(H) #2023_12_11_Time_9_ms_(63.06%)_Space_20.5_MB_(87.90%) | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* class TreeNode { | ||
* public $val = null; | ||
* public $left = null; | ||
* public $right = null; | ||
* function __construct($val = 0, $left = null, $right = null) { | ||
* $this->val = $val; | ||
* $this->left = $left; | ||
* $this->right = $right; | ||
* } | ||
* } | ||
*/ | ||
class Solution { | ||
/** | ||
* @param TreeNode $root | ||
* @return Integer | ||
*/ | ||
function maxDepth($root) { | ||
return $this->findDepth($root, 0); | ||
} | ||
|
||
private function findDepth($node, $currentDepth) { | ||
if ($node == null) { | ||
return 0; | ||
} | ||
$currentDepth++; | ||
return 1 | ||
+ max($this->findDepth($node->left, $currentDepth), $this->findDepth($node->right, $currentDepth)); | ||
} | ||
} |
38 changes: 38 additions & 0 deletions
38
src/main/php/g0101_0200/s0104_maximum_depth_of_binary_tree/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,38 @@ | ||
104\. Maximum Depth of Binary Tree | ||
|
||
Easy | ||
|
||
Given the `root` of a binary tree, return _its maximum depth_. | ||
|
||
A binary tree's **maximum depth** is the number of nodes along the longest path from the root node down to the farthest leaf node. | ||
|
||
**Example 1:** | ||
|
||
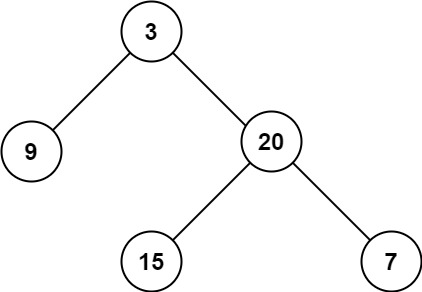 | ||
|
||
**Input:** root = [3,9,20,null,null,15,7] | ||
|
||
**Output:** 3 | ||
|
||
**Example 2:** | ||
|
||
**Input:** root = [1,null,2] | ||
|
||
**Output:** 2 | ||
|
||
**Example 3:** | ||
|
||
**Input:** root = [] | ||
|
||
**Output:** 0 | ||
|
||
**Example 4:** | ||
|
||
**Input:** root = [0] | ||
|
||
**Output:** 1 | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the tree is in the range <code>[0, 10<sup>4</sup>]</code>. | ||
* `-100 <= Node.val <= 100` |
61 changes: 61 additions & 0 deletions
61
...p/g0101_0200/s0105_construct_binary_tree_from_preorder_and_inorder_traversal/Solution.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,61 @@ | ||
<?php | ||
|
||
namespace leetcode\g0101_0200\s0105_construct_binary_tree_from_preorder_and_inorder_traversal; | ||
|
||
// #Medium #Top_100_Liked_Questions #Top_Interview_Questions #Array #Hash_Table #Tree #Binary_Tree | ||
// #Divide_and_Conquer #Data_Structure_II_Day_15_Tree #Big_O_Time_O(N)_Space_O(N) | ||
// #2023_12_11_Time_14_ms_(63.33%)_Space_21.1_MB_(93.33%) | ||
|
||
use leetcode\com_github_leetcode\TreeNode; | ||
|
||
/** | ||
* Definition for a binary tree node. | ||
* class TreeNode { | ||
* public $val = null; | ||
* public $left = null; | ||
* public $right = null; | ||
* function __construct($val = 0, $left = null, $right = null) { | ||
* $this->val = $val; | ||
* $this->left = $left; | ||
* $this->right = $right; | ||
* } | ||
* } | ||
*/ | ||
class Solution { | ||
private $j; | ||
private $map; | ||
|
||
function __construct() { | ||
$this->j = 0; | ||
$this->map = array(); | ||
} | ||
|
||
function get($key) { | ||
return $this->map[$key]; | ||
} | ||
|
||
private function answer($preorder, $inorder, $start, $end) { | ||
if ($start > $end || $this->j > count($preorder)) { | ||
return null; | ||
} | ||
$value = $preorder[$this->j++]; | ||
$index = $this->get($value); | ||
$node = new TreeNode($value); | ||
$node->left = $this->answer($preorder, $inorder, $start, $index - 1); | ||
$node->right = $this->answer($preorder, $inorder, $index + 1, $end); | ||
return $node; | ||
} | ||
|
||
/** | ||
* @param Integer[] $preorder | ||
* @param Integer[] $inorder | ||
* @return TreeNode | ||
*/ | ||
function buildTree($preorder, $inorder) { | ||
$this->j = 0; | ||
for ($i = 0; $i < count($preorder); $i++) { | ||
$this->map[$inorder[$i]] = $i; | ||
} | ||
return $this->answer($preorder, $inorder, 0, count($preorder) - 1); | ||
} | ||
} |
29 changes: 29 additions & 0 deletions
29
..._0200/s0105_construct_binary_tree_from_preorder_and_inorder_traversal/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
105\. Construct Binary Tree from Preorder and Inorder Traversal | ||
|
||
Medium | ||
|
||
Given two integer arrays `preorder` and `inorder` where `preorder` is the preorder traversal of a binary tree and `inorder` is the inorder traversal of the same tree, construct and return _the binary tree_. | ||
|
||
**Example 1:** | ||
|
||
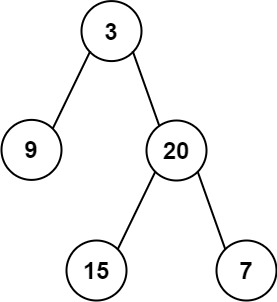 | ||
|
||
**Input:** preorder = [3,9,20,15,7], inorder = [9,3,15,20,7] | ||
|
||
**Output:** [3,9,20,null,null,15,7] | ||
|
||
**Example 2:** | ||
|
||
**Input:** preorder = [-1], inorder = [-1] | ||
|
||
**Output:** [-1] | ||
|
||
**Constraints:** | ||
|
||
* `1 <= preorder.length <= 3000` | ||
* `inorder.length == preorder.length` | ||
* `-3000 <= preorder[i], inorder[i] <= 3000` | ||
* `preorder` and `inorder` consist of **unique** values. | ||
* Each value of `inorder` also appears in `preorder`. | ||
* `preorder` is **guaranteed** to be the preorder traversal of the tree. | ||
* `inorder` is **guaranteed** to be the inorder traversal of the tree. |
Oops, something went wrong.